Frequently Asked Questions
On this page we'll provide answers to common questions that we frequently see in support.
Please feel free to reach out to us if you have any questions not answered here.
How can you programatically print ZPL code on a networked Zebra printer?
For a network connected Zebra printer the easiest way is to send the ZPL directly over a TCP socket. Zebra printers listen on TCP port 9100
by default.
On MacOS or Linux you can use the command line tool netcat / nc
to print a file containing ZPL code:
nc -N 192.168.1.234 9100 < my_label.zpl
Below is a Java sample to send a ZPL string (as returned by the API) to a printer:
String zplCode = ... // This should be the full ZPL code
try (Socket socket = new Socket("192.168.1.234", 9100)) {
OutputStream os = socket.getOutputStream();
os.write(zplCode.getBytes(StandardCharsets.US_ASCII));
os.flush();
} catch (IOException e) {
// Notify users of network errors here...
throw new RuntimeException(e);
}
How can you generate a barcode in HTML?
You can include a JavaScript library that renders a barcode.
Below is an example that adds a simple Code128 barcode using the JsBarcode library:
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/JsBarcode.all.min.js"></script>
<svg id="barcode"></svg>
<script>
JsBarcode("#barcode", "htmltozpl.com", {
format: "code128"
});
</script>
Will generate the following ZPL label:
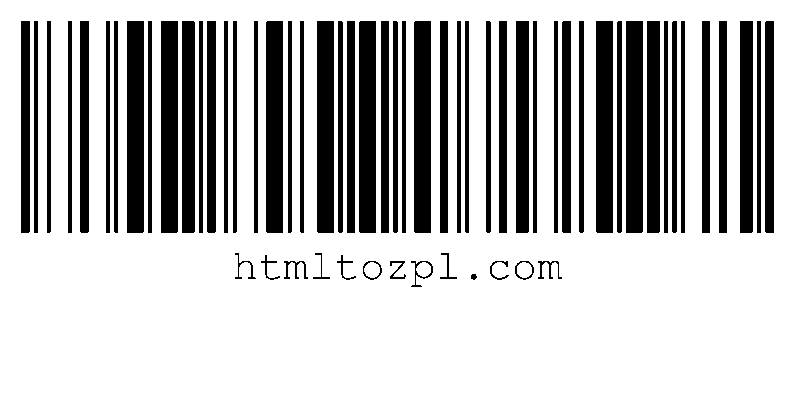
What is the best way to position elements accurately on a label?
We recommend to position elements using CSS absolute units, e.g. in
(inches) or mm
(millimeter). For example:
<style>
.box {
position: absolute;
box-sizing: border-box;
top: 1.5in;
left: 1in;
width: 2in;
height: 3in;
border: 5pt solid black;
padding: 5mm;
}
</style>
<div class="box">
This box is positioned using absolute units
and appears centered in a 4 x 6 label
</div>
This will render a label that looks like this:
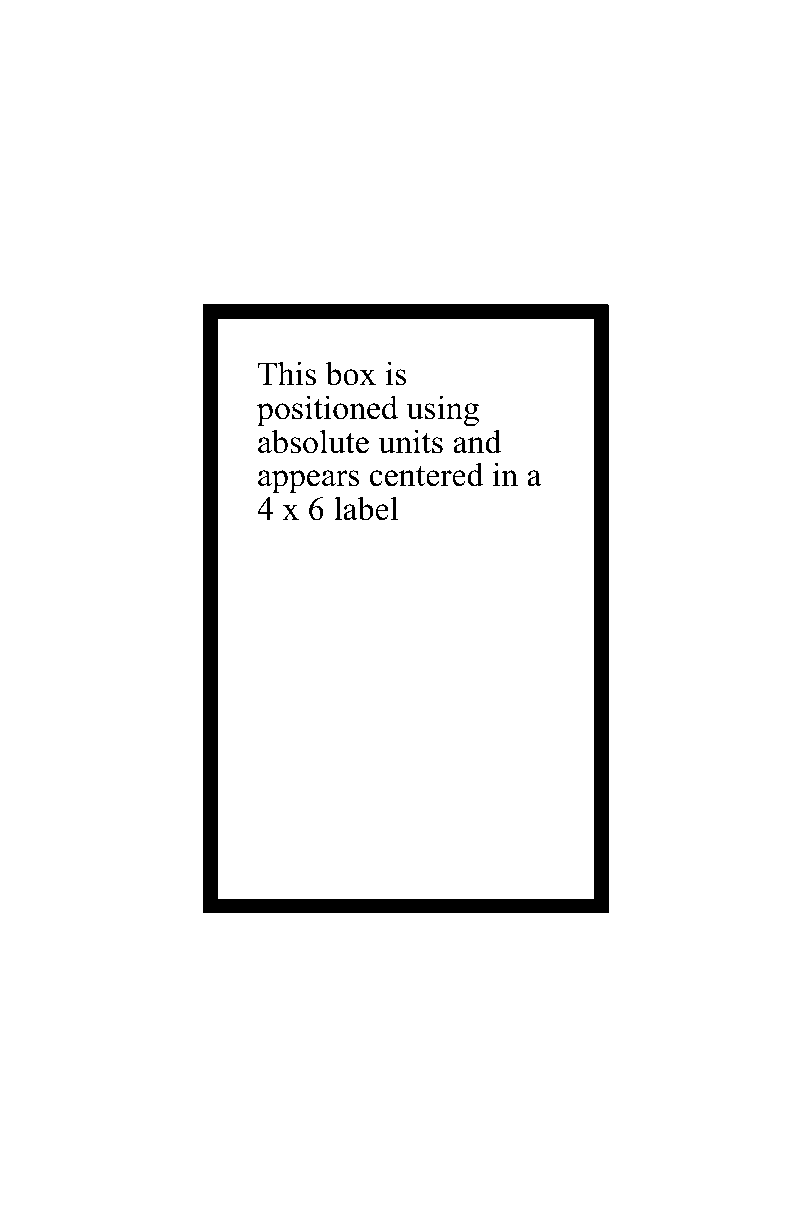
Is it possible to rotate a label?
Yes! You can use the CSS rotate function
to rotate any HTML elements. If you wrap the entire body in a div
with a rotation, the label will be rendered rotated.
For example:
<div style="transform: rotate(90deg);">
This will appear rotated 90°
</div>
How can you specifiy bleed values or a margin?
You can wrap your label's HTML within a <div>
container and add a padding.
Consider adding the box-sizing
style attribute, to prevent the padding from being
added to the box dimensions.
For example, to add a 0.5 inches margin around a 4"x6" label:
<div style="width: 4in; height: 6in; padding: 0.5in; box-sizing: border-box;">
... rest of label here ...
</div>
How can you print an image on the ZPL label?
You can just use the HTML <img>
tag directly to render an image:
<img src="https://www.example.org/test.png" style="width: 2in; height: 1in;" />
Note that this will trigger a network request on each invocation and you will have to host the
image publicly, so that our servers can access it.
If you would like to avoid that,
you can encode the image into base64 and include it directly in the URL. There are various online
service that can convert image files into base64 format,
for example base64-image.de,
or on Linux/MacOS you can use the base64
tool to encode a file on the command line.
<img src="data:image/png;base64, iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg=="
style="width: 2in; height: 1in;" />
Can I use a custom font on the ZPL label?
Yes! You can include web fonts via CSS.
Alternatively you can send us a short email and attach the font files (e.g. TTF or WOFF files). We'll make it available for use within the API.
I have another question or I found a bug! How can I get help?
Send us a quick email. We'll do our best to help out.